What Does It Mean When the Command Prompt Repeats a Line Over and Over Again Java

Welcome! If you lot desire to learn how to work with while loops in Python, then this commodity is for you lot.
While loops are very powerful programming structures that you can utilise in your programs to echo a sequence of statements.
In this commodity, you will learn:
- What while loops are.
- What they are used for.
- When they should be used.
- How they work behind the scenes.
- How to write a while loop in Python.
- What infinite loops are and how to interrupt them.
- What
while True
is used for and its full general syntax. - How to use a
break
statement to stop a while loop.
You will learn how while loops work backside the scenes with examples, tables, and diagrams.
Are you set up? Let's brainstorm. 🔅
🔹 Purpose and Use Cases for While Loops
Permit's beginning with the purpose of while loops. What are they used for?
They are used to repeat a sequence of statements an unknown number of times. This type of loop runs while a given condition is True
and it only stops when the condition becomes False
.
When we write a while loop, we don't explicitly define how many iterations will be completed, we only write the condition that has to be True
to proceed the process and False
to finish it.
💡 Tip: if the while loop condition never evaluates to Faux
, so nosotros will take an space loop, which is a loop that never stops (in theory) without external intervention.
These are some examples of real use cases of while loops:
- User Input: When we enquire for user input, we need to check if the value entered is valid. We can't possibly know in advance how many times the user volition enter an invalid input before the program can proceed. Therefore, a while loop would be perfect for this scenario.
- Search: searching for an element in a data construction is another perfect utilise example for a while loop because we tin can't know in advance how many iterations will be needed to observe the target value. For example, the Binary Search algorithm can be implemented using a while loop.
- Games: In a game, a while loop could be used to keep the main logic of the game running until the player loses or the game ends. Nosotros can't know in advance when this volition happen, and then this is some other perfect scenario for a while loop.
🔸 How While Loops Work
At present that you know what while loops are used for, let's see their principal logic and how they work behind the scenes. Here we accept a diagram:

Let'southward break this down in more detail:
- The process starts when a while loop is found during the execution of the program.
- The condition is evaluated to check if it'south
True
orFaux
. - If the status is
True
, the statements that vest to the loop are executed. - The while loop status is checked again.
- If the condition evaluates to
True
again, the sequence of statements runs again and the procedure is repeated. - When the status evaluates to
Simulated
, the loop stops and the program continues beyond the loop.
One of the nigh important characteristics of while loops is that the variables used in the loop status are not updated automatically. We have to update their values explicitly with our code to make sure that the loop will eventually stop when the condition evaluates to Fake
.
🔹 General Syntax of While Loops
Great. Now you lot know how while loops piece of work, so let's swoop into the code and see how you tin can write a while loop in Python. This is the basic syntax:

These are the main elements (in gild):
- The
while
keyword (followed by a space). - A condition to make up one's mind if the loop volition continue running or not based on its truth value (
True
orFalse
). - A colon (
:
) at the end of the first line. - The sequence of statements that will be repeated. This cake of code is called the "body" of the loop and information technology has to be indented. If a statement is non indented, it will not be considered part of the loop (please see the diagram below).
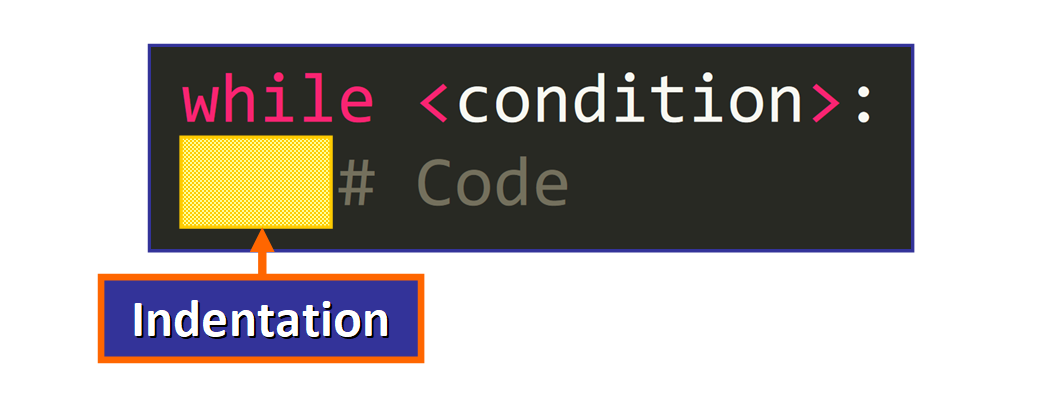
💡 Tip: The Python style guide (PEP 8) recommends using four spaces per indentation level. Tabs should merely be used to remain consistent with code that is already indented with tabs.
🔸 Examples of While Loops
Now that yous know how while loops work and how to write them in Python, let'south see how they work behind the scenes with some examples.
How a Basic While Loop Works
Here we have a basic while loop that prints the value of i
while i
is less than viii (i < 8
):
i = 4 while i < eight: print(i) i += ane
If we run the code, we see this output:
4 5 6 vii
Let's run into what happens behind the scenes when the code runs:
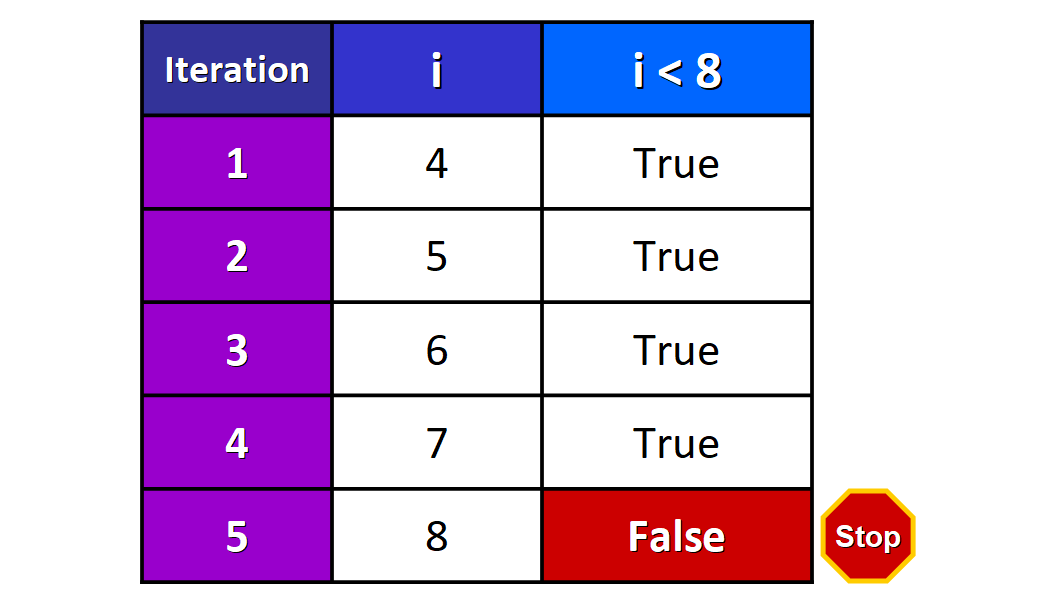
- Iteration 1: initially, the value of
i
is 4, so the statusi < viii
evaluates toTrue
and the loop starts to run. The value ofi
is printed (4) and this value is incremented by one. The loop starts again. - Iteration 2: now the value of
i
is 5, so the conditioni < viii
evaluates toTrue
. The body of the loop runs, the value ofi
is printed (5) and this valuei
is incremented past ane. The loop starts once again. - Iterations 3 and four: The aforementioned process is repeated for the tertiary and fourth iterations, and so the integers vi and vii are printed.
- Earlier starting the 5th iteration, the value of
i
is8
. At present the while loop statusi < viii
evaluates toFalse
and the loop stops immediately.
💡 Tip: If the while loop status is Imitation
before starting the start iteration, the while loop will not fifty-fifty start running.
User Input Using a While Loop
Now let's see an example of a while loop in a plan that takes user input. We will the input()
function to ask the user to enter an integer and that integer will merely be appended to list if information technology'southward even.
This is the code:
# Define the list nums = [] # The loop will run while the length of the # listing nums is less than iv while len(nums) < 4: # Ask for user input and store it in a variable every bit an integer. user_input = int(input("Enter an integer: ")) # If the input is an even number, add it to the list if user_input % 2 == 0: nums.append(user_input)
The loop condition is len(nums) < 4
, so the loop will run while the length of the list nums
is strictly less than 4.
Let's analyze this program line by line:
- Nosotros start by defining an empty listing and assigning it to a variable called
nums
.
nums = []
- And then, we define a while loop that will run while
len(nums) < 4
.
while len(nums) < iv:
- We ask for user input with the
input()
function and shop it in theuser_input
variable.
user_input = int(input("Enter an integer: "))
💡 Tip: Nosotros need to convert (bandage) the value entered by the user to an integer using the int()
role before assigning information technology to the variable considering the input()
function returns a cord (source).
- We check if this value is fifty-fifty or odd.
if user_input % two == 0:
- If it's even, we append it to the
nums
list.
nums.suspend(user_input)
- Else, if information technology'south odd, the loop starts over again and the condition is checked to determine if the loop should proceed or not.
If we run this code with custom user input, we get the following output:
Enter an integer: iii Enter an integer: 4 Enter an integer: ii Enter an integer: 1 Enter an integer: seven Enter an integer: half dozen Enter an integer: iii Enter an integer: 4
This table summarizes what happens behind the scenes when the code runs:

💡 Tip: The initial value of len(nums)
is 0
because the list is initially empty. The last column of the table shows the length of the listing at the end of the current iteration. This value is used to check the condition earlier the adjacent iteration starts.
Equally you tin can see in the table, the user enters even integers in the second, third, sixth, and eight iterations and these values are appended to the nums
list.
Before a "ninth" iteration starts, the condition is checked over again but at present it evaluates to False
considering the nums
listing has four elements (length 4), so the loop stops.
If nosotros cheque the value of the nums
list when the process has been completed, we meet this:
>>> nums [iv, 2, six, iv]
Exactly what we expected, the while loop stopped when the condition len(nums) < four
evaluated to False
.
Now you know how while loops work behind the scenes and you've seen some practical examples, so let's dive into a fundamental chemical element of while loops: the status.
🔹 Tips for the Condition in While Loops
Earlier you start working with while loops, you should know that the loop condition plays a central role in the functionality and output of a while loop.

You must be very careful with the comparison operator that you choose considering this is a very common source of bugs.
For example, common errors include:
- Using
<
(less than) instead of<=
(less than or equal to) (or vice versa). - Using
>
(greater than) instead of>=
(greater than or equal to) (or vice versa).
This can affect the number of iterations of the loop and even its output.
Allow's come across an example:
If nosotros write this while loop with the status i < 9
:
i = vi while i < 9: print(i) i += 1
We see this output when the code runs:
6 7 8
The loop completes three iterations and it stops when i
is equal to 9
.
This table illustrates what happens behind the scenes when the code runs:
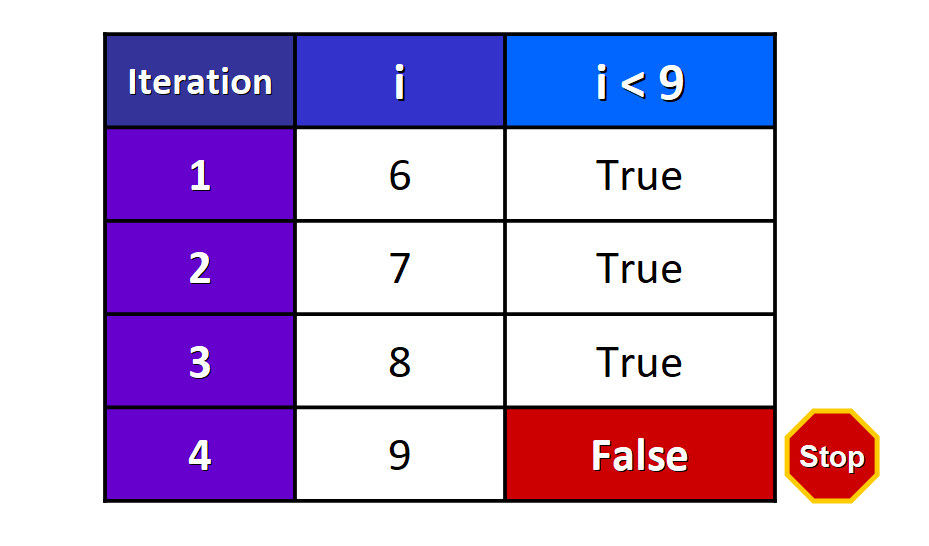
- Before the starting time iteration of the loop, the value of
i
is vi, then the conditioni < ix
isTrue
and the loop starts running. The value ofi
is printed and then it is incremented by one. - In the second iteration of the loop, the value of
i
is vii, so the statusi < nine
isTrue
. The body of the loop runs, the value ofi
is printed, and so it is incremented by 1. - In the third iteration of the loop, the value of
i
is 8, so the conditioni < 9
isTrue
. The trunk of the loop runs, the value ofi
is printed, and then it is incremented by ane. - The condition is checked again earlier a fourth iteration starts, but now the value of
i
is nine, and soi < 9
isImitation
and the loop stops.
In this case, we used <
as the comparison operator in the condition, simply what do you lot call back will happen if nosotros utilize <=
instead?
i = half dozen while i <= 9: print(i) i += one
We run across this output:
6 7 8 9
The loop completes 1 more iteration because at present we are using the "less than or equal to" operator <=
, so the condition is withal True
when i
is equal to 9
.
This table illustrates what happens backside the scenes:

Four iterations are completed. The condition is checked once again before starting a "fifth" iteration. At this indicate, the value of i
is x
, so the condition i <= 9
is False
and the loop stops.
🔸 Infinite While Loops
Now y'all know how while loops work, but what do you recollect volition happen if the while loop condition never evaluates to False
?
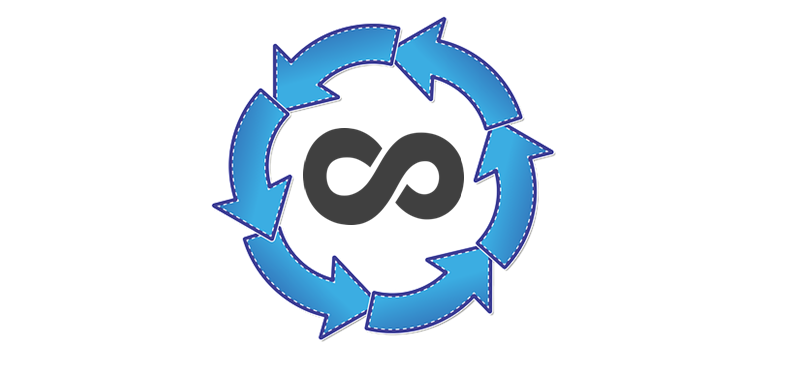
What are Infinite While Loops?
Recall that while loops don't update variables automatically (we are in accuse of doing that explicitly with our lawmaking). Then at that place is no guarantee that the loop volition stop unless nosotros write the necessary lawmaking to brand the condition False
at some signal during the execution of the loop.
If we don't do this and the condition always evaluates to True
, and so we will have an infinite loop, which is a while loop that runs indefinitely (in theory).
Space loops are typically the effect of a issues, merely they can too be caused intentionally when we want to repeat a sequence of statements indefinitely until a break
statement is found.
Let'south see these 2 types of space loops in the examples beneath.
💡 Tip: A issues is an fault in the plan that causes incorrect or unexpected results.
Example of Infinite Loop
This is an example of an unintentional space loop caused past a bug in the program:
# Define a variable i = 5 # Run this loop while i is less than 15 while i < 15: # Impress a message print("Howdy, Globe!")
Analyze this lawmaking for a moment.
Don't you lot find something missing in the body of the loop?
That's right!
The value of the variable i
is never updated (information technology's always 5
). Therefore, the status i < 15
is always Truthful
and the loop never stops.
If nosotros run this code, the output will be an "space" sequence of Hello, World!
messages because the body of the loop print("Hello, World!")
volition run indefinitely.
Hello, World! Hello, Globe! Hello, World! How-do-you-do, Globe! Howdy, World! How-do-you-do, World! Hello, World! Hullo, Earth! Hello, World! Hello, Earth! Hi, Globe! Hello, World! Hello, Globe! Howdy, World! Hello, Globe! Hello, World! Hello, World! Hi, World! . . . # Continues indefinitely
To stop the program, nosotros will demand to interrupt the loop manually by pressing CTRL + C
.
When we practice, we volition see a KeyboardInterrupt
error like to this one:

To prepare this loop, we volition need to update the value of i
in the torso of the loop to make sure that the condition i < 15
volition eventually evaluate to False
.
This is 1 possible solution, incrementing the value of i
by 2 on every iteration:
i = 5 while i < xv: print("Hello, Globe!") # Update the value of i i += 2
Great. Now you lot know how to fix space loops caused by a bug. You simply need to write code to guarantee that the condition will eventually evaluate to False
.
Let'due south start diving into intentional infinite loops and how they piece of work.
🔹 How to Make an Space Loop with While Truthful
We tin generate an infinite loop intentionally using while True
. In this case, the loop will run indefinitely until the procedure is stopped by external intervention (CTRL + C
) or when a break
statement is institute (you will larn more than about interruption
in only a moment).
This is the bones syntax:

Instead of writing a condition after the while
keyword, we just write the truth value directly to indicate that the condition will always be True
.
Here we take an example:
>>> while Truthful: print(0) 0 0 0 0 0 0 0 0 0 0 0 0 0 Traceback (most recent call last): File "<pyshell#ii>", line ii, in <module> print(0) KeyboardInterrupt
The loop runs until CTRL + C
is pressed, merely Python as well has a pause
statement that we can use directly in our code to cease this type of loop.
The pause
argument
This argument is used to stop a loop immediately. You should think of it every bit a red "stop sign" that y'all can use in your lawmaking to accept more control over the behavior of the loop.

According to the Python Documentation:
Thebreak
argument, like in C, breaks out of the innermost enclosingfor
orwhile
loop.
This diagram illustrates the bones logic of the break
argument:

break
statement This is the basic logic of the break
statement:
- The while loop starts only if the condition evaluates to
Truthful
. - If a
intermission
statement is plant at whatever betoken during the execution of the loop, the loop stops immediately. - Else, if
interruption
is not plant, the loop continues its normal execution and it stops when the condition evaluates toSimulated
.
We tin use break
to end a while loop when a condition is met at a particular point of its execution, so you will typically find information technology within a conditional statement, similar this:
while True: # Code if <status>: interruption # Code
This stops the loop immediately if the condition is True
.
💡 Tip: Yous tin (in theory) write a break
statement anywhere in the body of the loop. It doesn't necessarily have to be part of a conditional, but we commonly apply information technology to end the loop when a given condition is True
.
Here we have an example of break
in a while True
loop:
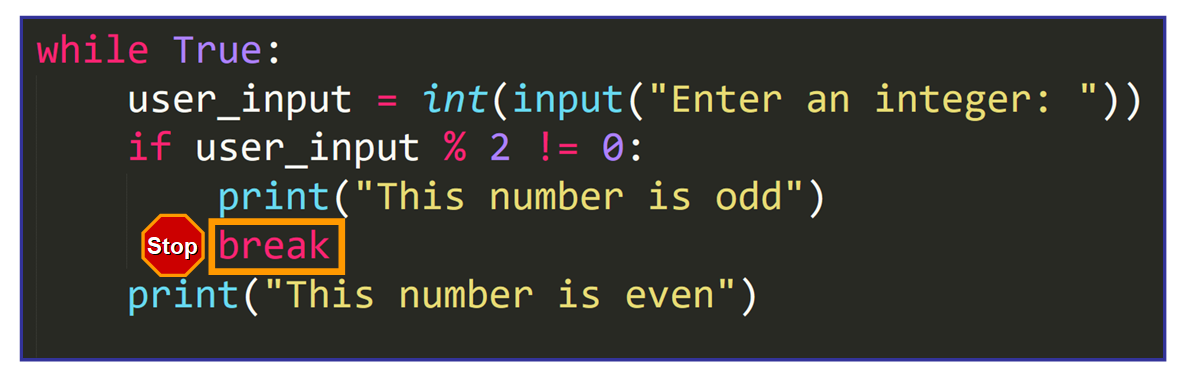
Allow's come across it in more than detail:
The commencement line defines a while True
loop that will run indefinitely until a pause
statement is found (or until it is interrupted with CTRL + C
).
while True:
The second line asks for user input. This input is converted to an integer and assigned to the variable user_input
.
user_input = int(input("Enter an integer: "))
The 3rd line checks if the input is odd.
if user_input % 2 != 0:
If information technology is, the bulletin This number is odd
is printed and the break
statement stops the loop immediately.
impress("This number of odd") break
Else, if the input is even , the bulletin This number is even
is printed and the loop starts again.
print("This number is even")
The loop will run indefinitely until an odd integer is entered because that is the just way in which the break
argument volition be found.
Here we take an instance with custom user input:
Enter an integer: 4 This number is even Enter an integer: half-dozen This number is fifty-fifty Enter an integer: viii This number is even Enter an integer: 3 This number is odd >>>
🔸 In Summary
- While loops are programming structures used to repeat a sequence of statements while a condition is
True
. They stop when the condition evaluates toFaux
. - When you write a while loop, yous need to make the necessary updates in your code to make sure that the loop will somewhen stop.
- An infinite loop is a loop that runs indefinitely and it merely stops with external intervention or when a
break
argument is found. - You can end an infinite loop with
CTRL + C
. - Yous tin generate an infinite loop intentionally with
while True
. - The
break
argument tin can be used to stop a while loop immediately.
I actually hope you liked my article and found it helpful. Now you know how to work with While Loops in Python.
Follow me on Twitter @EstefaniaCassN and if y'all want to learn more about this topic, check out my online course Python Loops and Looping Techniques: Beginner to Avant-garde.
Learn to lawmaking for free. freeCodeCamp's open source curriculum has helped more than 40,000 people go jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-while-loop-tutorial/
0 Response to "What Does It Mean When the Command Prompt Repeats a Line Over and Over Again Java"
Post a Comment